Java Core in Practice: 120+ Exercises and Quizzes for 2025
- Description
- Curriculum
- FAQ
- Reviews
Welcome to the World of Java Programming!
Welcome to our Java Core Course! We are happy to have you here as you begin your journey into programming or grow your skills. This course is perfect for everyone—whether you’re just starting, thinking about switching careers, or simply curious about coding. You don’t need a computer science degree to join—just a desire to learn.
Why Take This Course?
At ITER Academy, we have already taught over 1,000 students in classrooms. Now, we are bringing all that knowledge and experience online, so more people can benefit. Your teacher, Andrii Partola, is a Senior Java Engineer who works with Java every day. He uses Java to create powerful and complex programs for real companies. He has taken his real-world experience and turned it into lessons that are easy to understand and fun to follow.
Who Can Learn Java with Us?
This course is made for everyone.
-
Complete beginners: Even if you’ve never written a single line of code, this course will guide you step by step.
-
Career changers: If you want to start a new career in tech, this course will give you the tools you need.
-
Anyone curious about programming: If you’ve always wanted to try coding, this is a great place to start.
Learn with Real-Life Examples
Learning is easier when you can see how things work. That’s why this course is full of real-life examples. You’ll see how Java is used to solve problems, so you can understand not just what you’re learning, but also why it’s useful. These examples will help you remember things better and prepare you to use Java on your own.
Quizzes to Check Your Knowledge
To make sure you really understand the material, this course includes quizzes after many lessons. These quizzes will help you review important ideas and see how much you’ve learned. They’re a fun way to test yourself and feel confident before moving on to the next topic.
Practice Coding with Exercises
The best way to learn programming is by writing code yourself. That’s why this course has lots of coding exercises. These exercises give you a chance to practice what you’ve learned and solve problems like a real programmer. By doing this, you’ll get better and more confident with every lesson.
Lessons Designed for Success
We didn’t just create this course from scratch. We studied many other popular courses to learn what works best. Every lesson in this course is carefully organized, so it’s easy to follow and makes sense step by step. Each lesson is packed with useful information, so you learn quickly without wasting time.
Free Access to All Code
All the code written in this course is available on GitHub. You can download it, use it, and practice with it whenever you need. This means you’ll always have access to examples to help you as you keep learning.
Why Choose ITER Academy?
-
Practical knowledge: Learn the skills that are used in real programming jobs.
-
Simple and clear lessons: No confusing words or hard-to-follow explanations.
-
Step-by-step structure: Each lesson builds on the last one, so you never feel lost.
-
Experienced teacher: Learn from a professional Java engineer with years of experience.
Start Learning Today
With easy-to-follow lessons, plenty of examples, quizzes, and exercises, this course gives you everything you need to become confident in Java programming. Join us today and let’s start your journey into the world of coding together!
-
1Introduction to the courseVideo lesson
Overview of course and what will be covered
Goals and objectives of the course
Prerequisites and recommended background knowledge
Course materials and resources
Introduction to the Java programming language and its importance in the industry
-
2Setting up the development environment (Windows)Video lesson
Installing the Java Development Kit (JDK)
Configuring the JDK and setting the JAVA_HOME environment variable
-
3Setting up the development environment (macOS)Video lesson
Installing the Java Development Kit (JDK)
-
4Troubleshooting Common Setup IssuesText lesson
Common errors that may occur while setting up the development environment
How to troubleshoot and resolve these errors
-
5Creating and Running a Simple Java Program (Windows)Video lesson
Creating a new Java file
Understanding the structure of a Java program and the role of the main method
Writing code for a simple console program
Compiling .java files to bytecode
Running the program from the command line
-
6Creating and Running a Simple Java Program (macOS)Video lesson
Creating a new Java file
Understanding the structure of a Java program and the role of the main method
Writing code for a simple console program
Compiling .java files to bytecode
Running the program from the command line
-
7Introduction to IDEs and its importanceVideo lesson
Definition and purpose of IDEs
Comparison of IDEs to text editors
Importance of using an IDE in Java development
Popular IDEs for Java development
-
8Setting up an IDEVideo lesson
Downloading and installing IntelliJ IDEA
Creating a new project in the IntelliJ IDEA
Writing and executing a simple Java program
-
9Data types and variables - IntroductionVideo lesson
Explanation of what data types and variables are
Importance of understanding data types and variables in programming
-
10Primitive Data TypesVideo lesson
Explanation of primitive data types and their uses
Integer data types (byte, short, int, long)
Floating-point data types (float, double)
Character and boolean data types
Demonstration of how to declare and initialize variables of different primitive data types
Casting
-
11Primitive Data TypesQuiz
-
12Non-Primitive Data TypesVideo lesson
Explanation of non-primitive data types (String, arrays, classes)
Comparison of primitive and non-primitive data types
Demonstration of how to declare and initialize variables of non-primitive data types
-
13Non-Primitive Data TypesQuiz
This quiz covers the fundamental concepts of non-primitive data types in Java, focusing on Strings, arrays, and classes, as well as differences between primitive and non-primitive types.
-
14Variable Naming Conventions and ScopeVideo lesson
Explanation of variable naming conventions in Java
Explanation of variable scope
Demonstration of how variable scope affects program behavior
-
15Variable Naming Conventions and ScopeQuiz
This quiz covers the key concepts of variable naming conventions and scope in Java, ensuring a better understanding of best practices for variable names and the different scopes that affect variable accessibility.
-
16Writing to the consoleVideo lesson
Using the System.out.print() and System.out.println() methods to write to the console
Examples of writing different data types to the console
Formatting output with printf() method
-
17Writing to the consoleQuiz
This quiz covers the essential concepts of writing to the console in Java, focusing on the use of System.out.print(), System.out.println(), and System.out.printf() methods.
-
18Writing to the consoleQuiz
-
19Reading from the consoleVideo lesson
Using the Scanner class to read input from the console
Examples of reading different data types from the console
-
20Reading from the consoleQuiz
This quiz covers the essential concepts of reading input from the console using the Scanner class in Java, focusing on correct usage and exception handling.
-
21OperatorsVideo lesson
Arithmetic operators (e.g. +, -, *, /)
Comparison operators (e.g. ==, !=, >, <)
Logical operators (e.g. &&, ||, !)
Assignment operators (e.g. =, +=, -=)
-
22OperatorsQuiz
This quiz covers the essential concepts of operators in Java, focusing on arithmetic, comparison, logical, and assignment operators.
-
23OperatorsQuiz
-
24Operator precedenceVideo lesson
Understanding operator precedence in expressions
Examples of how operator precedence affects the outcome of an expression
-
25Operator precedenceQuiz
This quiz covers the essential concepts of operator precedence in Java, ensuring a better understanding of how different operators are prioritized in expressions.
-
26Common pitfalls and best practicesVideo lesson
Common mistakes when using operators and expressions
Best practices for writing clear and efficient code using operators and expressions
-
27Common pitfalls and best practicesQuiz
This quiz covers common pitfalls and best practices in Java programming, focusing on correct usage of operators, proper string declaration, order of operations, and improving code readability and maintainability.
-
28if-else StatementsVideo lesson
Syntax and usage of if-else statements
Example of if-else statements in Java code
Nesting of if-else statements
-
29if-else StatementsQuiz
This quiz covers the essential concepts of if-else statements in Java, focusing on correct syntax, nesting, and if-else ladder usage.
-
30if-else StatementsQuiz
-
31Switch StatementsVideo lesson
Syntax and usage of switch statements
Example of switch statements in Java code
Differences between switch and if-else statements
-
32Switch StatementsQuiz
This quiz covers the essential concepts of switch statements in Java, focusing on correct syntax, usage, and differences from if-else statements.
-
33Switch StatementsQuiz
-
34LoopsVideo lesson
Types of loops in Java (for, while, do-while)
Syntax and usage of each loop type
Examples of loops in Java code
Usage of break and continue
Tips for efficient looping
-
35LoopsQuiz
This quiz covers the essential concepts of loops in Java, focusing on for, while, and do-while loops, as well as the break and continue statements.
-
36Loops - forQuiz
-
37Loops - whileQuiz
-
38Loops - do-whileQuiz
-
39Loops - FactorialQuiz
-
40Methods in JavaVideo lesson
Definition of a method in Java
Syntax of declaring a method in Java
Types of methods (void, non-void)
Defining parameters for a method
Return types of methods
Method overloading
Method invocation
Parameter passing mechanism in Java
Recursive methods
Varargs in the methods
-
41Methods in JavaQuiz
This quiz covers the essential concepts of methods in Java, focusing on declaration, invocation, overloading, recursion, and varargs.
-
42Methods in Java - sum numbersQuiz
-
43ArraysVideo lesson
Creating and initializing arrays
Accessing and modifying array elements
Iterating through arrays
Common methods for arrays (length, sort, etc.)
Multidimensional arrays
-
44ArraysQuiz
This quiz covers the essential concepts of arrays in Java, focusing on declaration, initialization, accessing elements, modifying elements, common methods, and multidimensional arrays.
-
45Arrays - Initialize an arrayQuiz
-
46Arrays - Print all elementsQuiz
-
47Arrays - Get first elementQuiz
-
48Arrays - Initialize a two-dimensional arrayQuiz
-
49Arrays - Print all elements of the two-dimensional arrayQuiz
-
50Strings in JavaVideo lesson
Overview of the concept of strings in Java
Differences between string literals and string objects
-
51Strings in JavaQuiz
This quiz covers the essential concepts of strings in Java, focusing on creation, comparison, and important methods such as length() and equals().
-
52Strings in JavaQuiz
-
53String ObjectsVideo lesson
String methods such as length(), charAt(), indexOf()
Immutability of strings and its implications
-
54String ObjectsQuiz
This quiz covers the essential concepts of string objects in Java, focusing on common methods, immutability, and string interning.
-
55String ObjectsQuiz
-
56String ConcatenationVideo lesson
Using the + operator for concatenation
Using the StringBuilder and StringBuffer classes for concatenation
-
57String ConcatenationQuiz
This quiz covers the essential concepts of string concatenation in Java, focusing on the + operator, StringBuilder, and StringBuffer classes.
-
58String ConcatenationQuiz
-
59String ComparisonVideo lesson
Compare strings using the equals() and equalsIgnoreCase() methods
Compare strings using the == operator and the compareTo() method
-
60String ComparisonQuiz
This quiz covers the essential concepts of string comparison in Java, focusing on the equals(), equalsIgnoreCase(), ==, and compareTo() methods.
-
61String ComparisonQuiz
-
62String formatting using the String.format() methodVideo lesson
Syntax and usage of the String.format() method
Examples of formatting strings using placeholders and format specifiers
-
63String formatting using the String.format() methodQuiz
This quiz covers the essential concepts of string formatting using the String.format() method in Java, focusing on format specifiers and their usage.
-
64String formatting using the String.format() methodQuiz
-
65String PoolVideo lesson
Understanding of string pool concept
How string pool works in Java
-
66String PoolQuiz
This quiz covers the essential concepts of the string pool in Java, focusing on string creation, comparison, and the use of the intern() method.
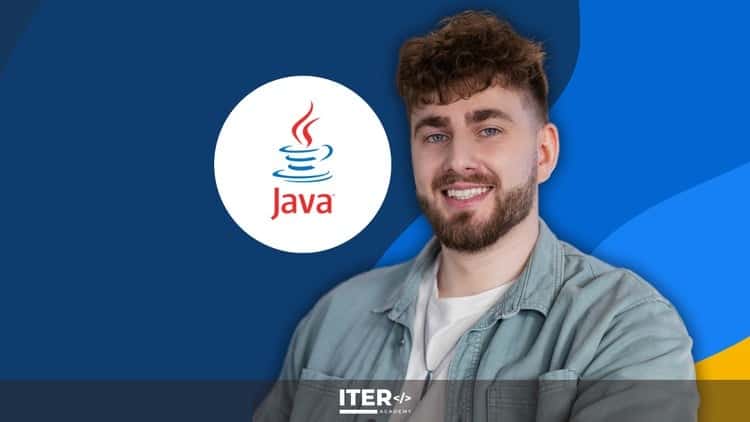