Java OOP: Object Oriented Programming with Exercises - 2025
- Description
- Curriculum
- FAQ
- Reviews
Welcome to the World of Java Programming!
Welcome to our Java Object Oriented Programming Course! We are happy to have you here as you begin your journey into programming or grow your skills. This course is perfect for everyone—whether you’re just starting, thinking about switching careers, or simply curious about coding. You don’t need a computer science degree to join—just a desire to learn.
Why Take This Course?
At ITER Academy, we have already taught over 1,000 students in classrooms. Now, we are bringing all that knowledge and experience online, so more people can benefit. Your teacher, Andrii Partola, is a Senior Java Engineer who works with Java every day. He uses Java to create powerful and complex programs for real companies. He has taken his real-world experience and turned it into lessons that are easy to understand and fun to follow.
Who Can Learn Java with Us?
This course is made for everyone.
-
Complete beginners: Even if you’ve never written a single line of code, this course will guide you step by step.
-
Career changers: If you want to start a new career in tech, this course will give you the tools you need.
-
Anyone curious about programming: If you’ve always wanted to try coding, this is a great place to start.
Learn with Real-Life Examples
Learning is easier when you can see how things work. That’s why this course is full of real-life examples. You’ll see how Java is used to solve problems, so you can understand not just what you’re learning, but also why it’s useful. These examples will help you remember things better and prepare you to use Java on your own.
Quizzes to Check Your Knowledge
To make sure you really understand the material, this course includes quizzes after many lessons. These quizzes will help you review important ideas and see how much you’ve learned. They’re a fun way to test yourself and feel confident before moving on to the next topic.
Practice Coding with Exercises
The best way to learn programming is by writing code yourself. That’s why this course has lots of coding exercises. These exercises give you a chance to practice what you’ve learned and solve problems like a real programmer. By doing this, you’ll get better and more confident with every lesson.
Lessons Designed for Success
We didn’t just create this course from scratch. We studied many other popular courses to learn what works best. Every lesson in this course is carefully organized, so it’s easy to follow and makes sense step by step. Each lesson is packed with useful information, so you learn quickly without wasting time.
Free Access to All Code
All the code written in this course is available on GitHub. You can download it, use it, and practice with it whenever you need. This means you’ll always have access to examples to help you as you keep learning.
Why Choose ITER Academy?
-
Practical knowledge: Learn the skills that are used in real programming jobs.
-
Simple and clear lessons: No confusing words or hard-to-follow explanations.
-
Step-by-step structure: Each lesson builds on the last one, so you never feel lost.
-
Experienced teacher: Learn from a professional Java engineer with years of experience.
Start Learning Today
With easy-to-follow lessons, plenty of examples, quizzes, and exercises, this course gives you everything you need to become confident in Java programming. Join us today and let’s start your journey into the world of coding together!
-
4ObjectsVideo lesson
Definition and explanation of an object in Java
Creating an object from a class
Understanding the relationship between classes and objects
Using the dot operator to access object methods and variables
Understanding the difference between an object's reference and its value
-
5ObjectsQuiz
This quiz covers the essential concepts of objects in Java, focusing on object creation, the relationship between classes and objects, constructors, and accessing object fields.
-
6ObjectsQuiz
-
7Advanced Classes and ObjectsVideo lesson
'static' keyword
Access modifiers
Initialization blocks
Nested classes
Getters and setters
-
8Advanced Classes and ObjectsQuiz
This quiz covers advanced concepts of classes and objects in Java, including the use of the static keyword, access modifiers, initialization blocks, nested classes, and getters and setters.
-
9Advanced Classes and Objects - 'static' keywordQuiz
-
10Advanced Classes and Objects - Access modifiersQuiz
-
11Advanced Classes and Objects - Initialization blocksQuiz
-
12Advanced Classes and Objects - Nested classesQuiz
-
13Advanced Classes and Objects - Getters and settersQuiz
-
14ConstructorsVideo lesson
Definition and explanation of constructors in Java
Types of constructors: default and parameterized
'this' keyword
How constructors are used when creating objects
-
15ConstructorsQuiz
This quiz covers the essential concepts of constructors in Java, focusing on their definition, usage, types, overloading, and chaining.
-
16ConstructorsQuiz
-
17InterfacesVideo lesson
Overview of interfaces in Java
Defining an interface
Implementing an interface
Extending an interface
Default and static methods in interfaces
-
18InterfacesQuiz
This quiz covers the essential concepts of interfaces in Java, focusing on their definition, implementation, multiple inheritance, default methods, and static methods.
-
19InterfacesQuiz
-
20Abstract ClassesVideo lesson
Overview of abstract classes in Java
Defining an abstract class
Extending an abstract class
Abstract methods and concrete methods
Abstract classes vs concrete classes
-
21Abstract ClassesQuiz
This quiz covers the essential concepts of abstract classes in Java, focusing on their definition, usage, and the differences between abstract and concrete methods.
-
22Abstract ClassesQuiz
-
23Combining Interfaces and Abstract ClassesVideo lesson
Differences between interfaces and abstract classes
When to use interfaces and when to use abstract classes
Extending an abstract class and implementing an interface
Differences between "implements" and "extends"
-
24Combining Interfaces and Abstract ClassesQuiz
This quiz covers the essential concepts of combining interfaces and abstract classes in Java, focusing on their differences, usage, and the implementation of both in a single class.
-
25Combining Interfaces and Abstract ClassesQuiz
-
26InheritanceVideo lesson
Definition and explanation of inheritance in OOP
Examples of inheritance in real-world scenarios
How inheritance allows for code reuse and organization
Extending classes in Java
Understanding the relationship between parent and child classes
Overriding methods in child classes
Understanding the use of the "super" keyword
Understanding the "is-a" relationship in inheritance
-
27InheritanceQuiz
This quiz covers the essential concepts of inheritance in Java, focusing on class hierarchies, method overriding, the super keyword, and access control.
-
28InheritanceQuiz
-
29PolymorphismVideo lesson
Definition and explanation of polymorphism in OOP
How polymorphism allows for flexibility and dynamic behavior in code
Understanding the difference between overloading and overriding methods
Examples of polymorphism in use, such as with the "instanceof" operator
-
30PolymorphismQuiz
This quiz covers the essential concepts of polymorphism in Java, focusing on method overloading, method overriding, the instanceof operator, and dynamic dispatch.
-
31PolymorphismQuiz
-
32Advanced Inheritance and PolymorphismVideo lesson
Understanding the use of the "final" keyword in classes and methods
Understanding the use of the "protected" access modifier
Understanding the difference between "composition" and "inheritance"
Understanding the use of inner classes and anonymous classes in polymorphism
Understanding the use of typecasting and downcasting
-
33Advanced Inheritance and PolymorphismQuiz
This quiz covers advanced concepts of inheritance and polymorphism in Java, focusing on the final keyword, protected access modifier, composition vs inheritance, inner and anonymous classes, typecasting, and downcasting.
-
34Advanced Inheritance and Polymorphism - the "protected" access modifierQuiz
-
35Advanced Inheritance and Polymorphism - "composition" and "inheritance"Quiz
-
36Advanced Inheritance and Polymorphism - the use of inner classes and anonymous classesQuiz
-
37EncapsulationVideo lesson
Definition of Encapsulation
Explanation of how encapsulation works
Explanation of public, private, protected and default access modifiers
Use of access modifiers in classes, methods and variables
Code examples of encapsulation in practice
-
38EncapsulationQuiz
This quiz covers the essential concepts of encapsulation in Java, focusing on access modifiers, getter and setter methods, and the benefits of encapsulation in software development.
-
39EncapsulationQuiz
-
40SOLID PrinciplesVideo lesson
Explanation of the acronym SOLID and its meaning
Definition and explanation of the Single Responsibility Principle
Definition and explanation of the Open-Closed Principle
Definition and explanation of the Liskov Substitution Principle
Definition and explanation of the Interface Segregation Principle
Definition and explanation of the Dependency Inversion Principle
-
41SOLID PrinciplesQuiz
This quiz covers the essential concepts of the SOLID principles in Java, focusing on each principle's definition, benefits, and application in software development.
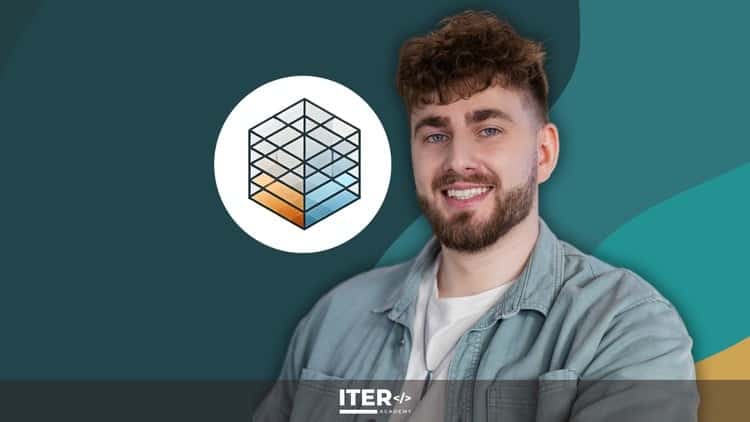